Learn how to build a data visualization dashboard using Python and Dash. Create interactive and dynamic data visualizations to explore and communicate insights effectively. Step-by-step tutorial with code examples using Dash, pandas, and Plotly. Master the art of building interactive dashboards and unleash the power of data visualization
Introduction:
In this tutorial, we will build a data visualization dashboard using Python and Dash. Data visualization is a powerful way to explore and communicate insights from data. Dash is a popular Python framework for building interactive web applications and dashboards. We will leverage the capabilities of Dash to create a dynamic and interactive data visualization dashboard. By the end of this tutorial, you will have a functional dashboard that allows users to explore and interact with data visualizations.
Prerequisites:
1. Basic understanding of Python programming.
2. Familiarity with data visualization concepts and libraries like Matplotlib or Plotly.
Step 1: Setting Up the Environment
Create a new directory for your project and navigate to it in a terminal or command prompt. Set up a virtual environment:
```
$ python -m venv data-visualization-env
```
Activate the virtual environment:
- On Windows:
```
$ data-visualization-env\Scripts\activate
```
- On macOS/Linux:
```
$ source data-visualization-env/bin/activate
```
Step 2: Installing Dependencies
Inside the activated virtual environment, install the necessary libraries:
```
$ pip install dash pandas plotly
```
Step 3: Data Preparation
Prepare your dataset or data source for visualization. Ensure that it is in a suitable format, such as a CSV file or a database.
Step 4: Writing the Code
Create a new Python file in your project directory, e.g., `data_visualization_dashboard.py`. Open the file in a text editor or IDE and follow along with the code below:
```python
import pandas as pd
import dash
import dash_core_components as dcc
import dash_html_components as html
import plotly.express as px
# Load the dataset
data = pd.read_csv('path/to/dataset.csv')
# Create the Dash app
app = dash.Dash(__name__)
# Define the layout
app.layout = html.Div([
dcc.Graph(
id='visualization',
figure=px.bar(data, x='x_column', y='y_column')
)
])
# Run the app
if __name__ == '__main__':
app.run_server(debug=True)
```
Step 5: Understanding the Code
- We import pandas for data manipulation, Dash for creating the app, dash_core_components and dash_html_components for building the layout, and plotly.express for data visualization.
- The dataset is loaded into a pandas DataFrame.
- The Dash app is created.
- The layout is defined using HTML components and a plotly.express chart.
- The app is run with the `run_server()` method.
Step 6: Running the Data Visualization Dashboard
Save the `data_visualization_dashboard.py` file and execute it from the command line:
```
$ python data_visualization_dashboard.py
```
The data visualization dashboard will be hosted locally, and you can access it by visiting the provided URL in your web browser.
Conclusion:
In this tutorial, we built a data visualization dashboard using Python and Dash. Dash provides a flexible and interactive environment for creating powerful data visualizations. You can further enhance the dashboard by incorporating additional components, interactive elements, or multiple visualizations. Start exploring and presenting your data with your own data visualization dashboard!
Support My Work with a Cup of Chai ! ☕
If you are located in India, I kindly request your support through a small contribution.
Please note that the UPI payment method is only available within India.
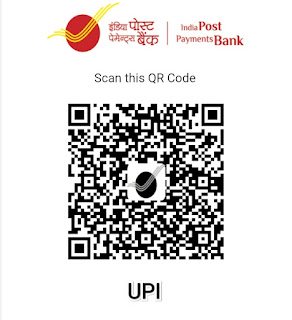
Accepted Payment Methods: Google Pay, PhonePe, PayTM, Amazonpay UPI
UPI ID :
haneen@postbank
If you are not located in India, you can still show your appreciation by sending a thank you or an Amazon gift card to the following email address:
websitehaneen@gmail.com
Wishing you a wonderful day!
HaneentheCREATE is now available in the Nas community (Nas Daily)! Become a member and join us