Learn how to build a password manager application in Python using cryptography. Safely store and retrieve passwords for different accounts. Encrypt and decrypt passwords using a chosen encryption key. Step-by-step tutorial with code examples.
Introduction:
In this tutorial, we will build a password manager application using Python. A password manager is a secure solution for storing and managing passwords for different accounts. We will learn how to encrypt and store passwords, retrieve and decrypt them when needed, and add features like password generation and secure storage using Python's cryptography library.
Prerequisites:
1. Basic understanding of Python programming.
2. Familiarity with encryption concepts and Python data structures.
Step 1: Setting Up the Environment
Create a new directory for your project and navigate to it in a terminal or command prompt. Create a virtual environment:
```
$ python -m venv password-manager-env
```
Activate the virtual environment:
- On Windows:
```
$ password-manager-env\Scripts\activate
```
- On macOS/Linux:
```
$ source password-manager-env/bin/activate
```
Step 2: Installing Dependencies
Inside the activated virtual environment, install the necessary libraries:
```
$ pip install cryptography
```
Step 3: Writing the Code
Create a new Python file in your project directory, e.g., `password_manager.py`. Open the file in a text editor or IDE and follow along with the code below:
```python
from cryptography.fernet import Fernet
import json
def generate_key():
key = Fernet.generate_key()
with open('key.key', 'wb') as key_file:
key_file.write(key)
def load_key():
with open('key.key', 'rb') as key_file:
key = key_file.read()
return key
def encrypt_password(key, password):
cipher = Fernet(key)
encrypted_password = cipher.encrypt(password.encode())
return encrypted_password
def decrypt_password(key, encrypted_password):
cipher = Fernet(key)
decrypted_password = cipher.decrypt(encrypted_password).decode()
return decrypted_password
def add_password(key, account, password):
encrypted_password = encrypt_password(key, password)
passwords = {}
try:
with open('passwords.json', 'r') as file:
passwords = json.load(file)
except FileNotFoundError:
pass
passwords[account] = encrypted_password
with open('passwords.json', 'w') as file:
json.dump(passwords, file)
def get_password(key, account):
with open('passwords.json', 'r') as file:
passwords = json.load(file)
encrypted_password = passwords.get(account)
if encrypted_password:
return decrypt_password(key, encrypted_password)
else:
return None
# Generate a new key (run only once)
# generate_key()
# Load the key
key = load_key()
# Add a password
account = 'example_account'
password = 'example_password'
add_password(key, account, password)
# Retrieve the password
retrieved_password = get_password(key, account)
print(f"Retrieved password for {account}: {retrieved_password}")
```
Step 4: Understanding the Code
- We import the `Fernet` class from the `cryptography.fernet` module, which provides encryption and decryption functionalities.
- The `generate_key()` function generates a new encryption key and stores it in a file called `key.key`.
- The `load_key()` function reads the encryption key from the `key.key` file.
- The `encrypt_password()` function encrypts a password using the provided encryption key.
- The `decrypt_password()` function decrypts an encrypted password using the encryption key.
- The `add_password()` function adds an account and its encrypted password to a JSON file called `passwords.json`.
- The `get_password()` function retrieves and decrypts a password for a given account from
the `passwords.json` file.
- In the example usage section, we generate a new key (commented out after the first run), load the key, add an example password, and then retrieve it.
Step 5: Running the Password Manager
Save the `password_manager.py` file and execute it from the command line:
```
$ python password_manager.py
```
The example password will be encrypted, added to the password manager, and then retrieved and decrypted, demonstrating the functionality of the tool.
Conclusion:
In this tutorial, we built a password manager application using Python's cryptography library. You can now securely store and retrieve passwords for different accounts. Enhance the tool by adding features like password generation, user authentication, and integrating with a database for secure storage. Keep your passwords safe and easily accessible with your custom password manager!
Support My Work with a Cup of Chai ! ☕
If you are located in India, I kindly request your support through a small contribution.
Please note that the UPI payment method is only available within India.
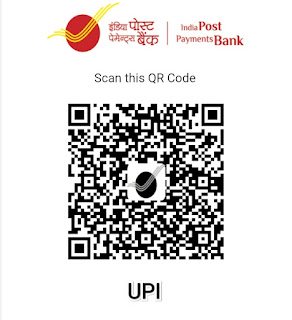
Accepted Payment Methods: Google Pay, PhonePe, PayTM, Amazonpay UPI
UPI ID :
haneen@postbank
If you are not located in India, you can still show your appreciation by sending a thank you or an Amazon gift card to the following email address:
websitehaneen@gmail.com
Wishing you a wonderful day!
HaneentheCREATE is now available in the Nas community (Nas Daily)! Become a member and join us