Introduction:
In this tutorial, we will build a currency converter application using Python. The application will allow users to convert between different currencies based on the current exchange rates. We will utilize third-party APIs and Python libraries such as requests and JSON to fetch and process the exchange rate data.
Prerequisites:
1. Basic understanding of Python programming.
2. Familiarity with working with APIs and JSON data.
Step 1: Setting Up the Environment
Create a new directory for your project and set up a virtual environment:
```
$ python -m venv currency-env
```
Activate the virtual environment:
- On Windows:
```
$ currency-env\Scripts\activate
```
- On macOS/Linux:
```
$ source currency-env/bin/activate
```
Step 2: Installing Dependencies
Inside the activated virtual environment, install the necessary libraries:
```
$ pip install requests
```
Step 3: Accessing Exchange Rate Data
Choose a reliable currency exchange rate API and sign up for an API key. Make sure the API provides current exchange rates for various currencies.
Step 4: Writing the Code
Create a new Python file in your project directory, e.g., `currency_converter.py`. Open the file in a text editor or IDE and follow along with the code below:
```python
import requests
def get_exchange_rate(base_currency, target_currency):
api_key = 'YOUR_API_KEY'
url = f'https://api.exchangerate-api.com/v4/latest/{base_currency}'
response = requests.get(url)
data = response.json()
exchange_rate = data['rates'][target_currency]
return exchange_rate
def convert_currency(amount, base_currency, target_currency):
exchange_rate = get_exchange_rate(base_currency, target_currency)
converted_amount = amount * exchange_rate
return converted_amount
# Example usage
amount = 100
base_currency = 'USD'
target_currency = 'EUR'
converted_amount = convert_currency(amount, base_currency, target_currency)
print(f'{amount} {base_currency} is equal to {converted_amount} {target_currency}')
```
Step 5: Understanding the Code
- We define a `get_exchange_rate()` function that fetches the exchange rate between a base currency and a target currency using the chosen API.
- We define a `convert_currency()` function that calculates the converted amount by multiplying the amount by the exchange rate.
- In the example usage section, we provide an amount, base currency, and target currency to convert. We call the `convert_currency()` function and print the converted amount.
Step 6: Running the Currency Converter
Save the `currency_converter.py` file and execute it from the command line:
```
$ python currency_converter.py
```
You should see the converted amount printed to the console.
Conclusion:
In this tutorial, we built a currency converter application using Python. By utilizing APIs and Python libraries, we were able to fetch exchange rate data and perform currency conversions. You can further enhance the application by adding more functionality, such as supporting multiple currencies or implementing a graphical user interface (GUI). Happy converting!
Support My Work with a Cup of Chai ! ☕
If you are located in India, I kindly request your support through a small contribution.
Please note that the UPI payment method is only available within India.
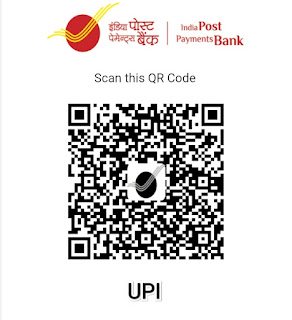
Accepted Payment Methods: Google Pay, PhonePe, PayTM, Amazonpay UPI
UPI ID :
haneen@postbank
If you are not located in India, you can still show your appreciation by sending a thank you or an Amazon gift card to the following email address:
websitehaneen@gmail.com
Wishing you a wonderful day!
HaneentheCREATE is now available in the Nas community (Nas Daily)! Become a member and join us