Unlock the power of Natural Language Processing (NLP) with Python. Learn text analysis, sentiment analysis, and more. Step-by-step tutorial with code examples.
Introduction:
In this beginner-friendly guide, we will dive into Natural Language Processing (NLP) using Python. NLP is a branch of artificial intelligence that focuses on the interaction between computers and human language. We will explore key concepts and practical examples to gain insights into text processing, sentiment analysis, and more.
Prerequisites:
1. Basic understanding of Python programming.
2. Familiarity with basic concepts of machine learning is a plus but not required.
Step 1: Setting Up the Environment
Start by creating a new directory for your project. Open a terminal or command prompt and navigate to the project directory. Create a virtual environment using the following command:
```
$ python -m venv nlp-env
```
Activate the virtual environment:
- On Windows:
```
$ nlp-env\Scripts\activate
```
- On macOS/Linux:
```
$ source nlp-env/bin/activate
```
Step 2: Installing Dependencies
Inside the activated virtual environment, install the necessary libraries:
```
$ pip install nltk
$ pip install spacy
```
Step 3: Preparing the Data
For this tutorial, we will use a sample dataset. Download and extract the dataset to your project directory.
Step 4: Writing the Code
Create a new Python file in your project directory, e.g., `nlp_processing.py`. Open the file in a text editor or integrated development environment (IDE) and follow along with the code below:
```python
import nltk
import spacy
# Load the dataset
dataset_path = 'path_to_dataset'
data = open(dataset_path, 'r').read()
# Tokenization using NLTK
tokens = nltk.word_tokenize(data)
# Part-of-speech tagging using NLTK
pos_tags = nltk.pos_tag(tokens)
# Named Entity Recognition (NER) using spaCy
nlp = spacy.load('en_core_web_sm')
doc = nlp(data)
ner_entities = [(ent.text, ent.label_) for ent in doc.ents]
# Sentiment Analysis using NLTK
from nltk.sentiment import SentimentIntensityAnalyzer
sid = SentimentIntensityAnalyzer()
sentiment_score = sid.polarity_scores(data)
# Print the results
print("Tokens:", tokens[:10])
print("POS Tags:", pos_tags[:10])
print("NER Entities:", ner_entities[:10])
print("Sentiment Score:", sentiment_score['compound'])
```
Step 5: Understanding the Code
- We import the required libraries: `nltk` for tokenization, part-of-speech tagging, and sentiment analysis, and `spacy` for named entity recognition.
- We load the dataset from the specified path.
- Tokenization: We use `nltk.word_tokenize()` to split the text into individual words (tokens).
- Part-of-speech Tagging: We use `nltk.pos_tag()` to assign grammatical tags to each token.
- Named Entity Recognition (NER): We use spaCy's pre-trained model (`en_core_web_sm`) to identify named entities in the text.
- Sentiment Analysis: We use the `SentimentIntensityAnalyzer` from NLTK to compute the sentiment score of the text.
- Finally, we print the results.
Step 6: Running the NLP Processing
Save the `nlp_processing.py` file and execute it from the command line:
```
$ python nlp_processing.py
```
You should see the tokens, POS tags, named entities, and sentiment score printed to the console.
Conclusion:
In this tutorial, we explored the fundamentals of Natural Language Processing (NLP) using Python. We learned how to tokenize text, assign part-of-speech tags, perform named entity recognition,
and analyze sentiment using popular libraries such as NLTK and spaCy. NLP has wide-ranging applications, from chatbots to language translation, and is an exciting field of study. Feel free to experiment further with different datasets and explore advanced NLP techniques to enhance your understanding. Happy exploring!
Support My Work with a Cup of Chai ! ☕
If you are located in India, I kindly request your support through a small contribution.
Please note that the UPI payment method is only available within India.
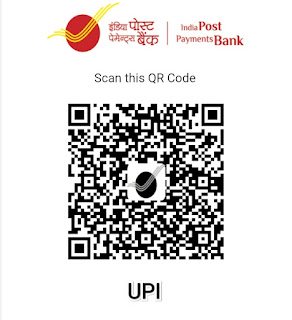
Accepted Payment Methods: Google Pay, PhonePe, PayTM, Amazonpay UPI
UPI ID :
haneen@postbank
If you are not located in India, you can still show your appreciation by sending a thank you or an Amazon gift card to the following email address:
websitehaneen@gmail.com
Wishing you a wonderful day!
HaneentheCREATE is now available in the Nas community (Nas Daily)! Become a member and join us